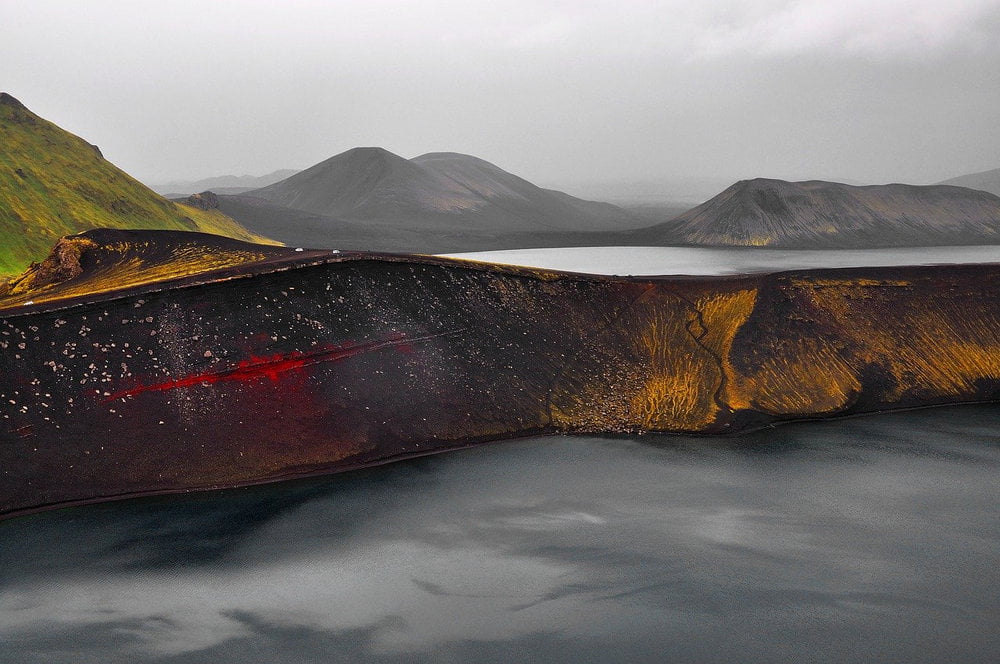
本文没有直接使用 StackExchange.Redis,而是使用 StackExchange.Redis.Extensions.Core 扩展。
目录
安装步骤
创建 ASP.NET Core 应用程序 StackExchangeRedisDemo
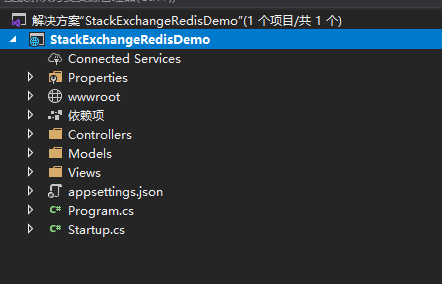
安装如下 Nuget 包
- StackExchange.Redis.Extensions.Core
- StackExchange.Redis.Extensions.AspNetCore
- StackExchange.Redis.Extensions.Newtonsoft
appsettings.json 添加如下配置
"Redis": {
"Password": "123456",
"AllowAdmin": true,
"Ssl": false,
"ConnectTimeout": 6000,
"ConnectRetry": 2,
"Database": 0,
"Hosts": [
{
"Host": "127.0.0.1",
"Port": "6379"
}
]
}
ConfigureServices 中注册 StackExchangeRedis 服务
public void ConfigureServices(IServiceCollection services)
{
var redisConfiguration = Configuration.GetSection("Redis").Get<RedisConfiguration>();
services.AddControllersWithViews();
services.AddStackExchangeRedisExtensions<NewtonsoftSerializer>(redisConfiguration);
}
使用演示
在 Models 文件夹中添加 Product 类
namespace StackExchangeRedisDemo.Models
{
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public double Price { get; set; }
}
}
注入 IRedisCacheClient
public class DemoController : Controller
{
private readonly IRedisCacheClient _redisCacheClient;
public DemoController(IRedisCacheClient redisCacheClient)
{
_redisCacheClient = redisCacheClient;
}
}
AddAsync 存储一个对象到 Redis
public async Task<IActionResult> Index()
{
var product = new Product()
{
Id = 1,
Name = "hand sanitizer",
Price = 100
};
bool isAdded = await _redisCacheClient.Db0.AddAsync("Product", product, DateTimeOffset.Now.AddMinutes(10));
return View();
}
使用 Redis Desktop Manager 查看
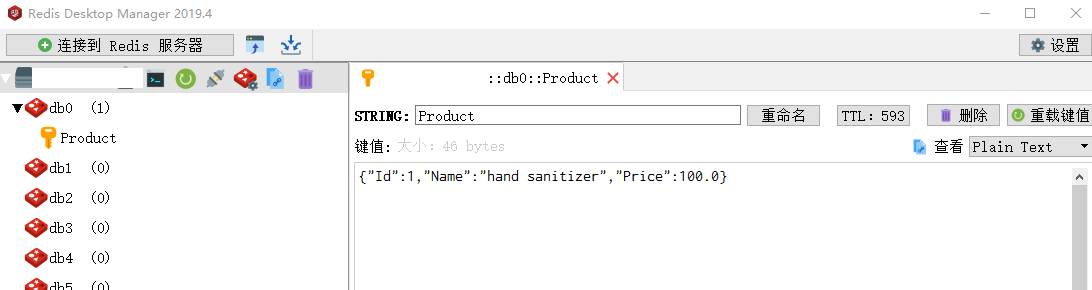
AddAllAsync 一次存储多个对象
public async Task<IActionResult> Index()
{
var values = new List<Tuple<string, Product>>
{
new Tuple<string, Product>("Product1", new Product()
{
Id = 1,
Name = "hand sanitizer 1",
Price = 100
}),
new Tuple<string, Product>("Product2",new Product()
{
Id = 2,
Name = "hand sanitizer 2",
Price = 200
}),
new Tuple<string, Product>("Product3", new Product()
{
Id = 3,
Name = "hand sanitizer 3",
Price = 300
})
};
await _redisCacheClient.Db0.AddAllAsync(values, DateTimeOffset.Now.AddMinutes(30));
return View();
}
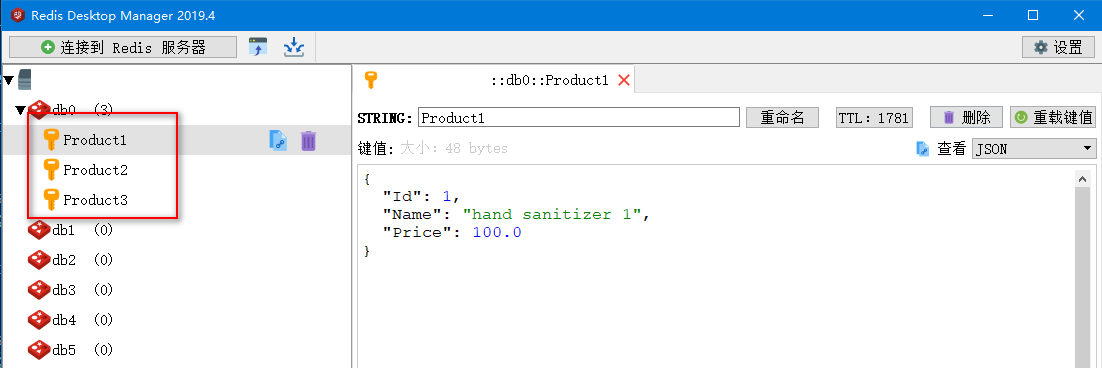
GetAsync 获取一个对象
public async Task<IActionResult> Index()
{
var productdata = await _redisCacheClient.Db0.GetAsync<Product>("Product");
return View();
}

GetAllAsync 一次获取多个对象
public async Task<IActionResult> Index()
{
List<string> allKeys = new List<string>()
{
"Product1","Product2","Product3"
};
var listofProducts = await _redisCacheClient.Db0.GetAllAsync<Product>(allKeys);
return View();
}
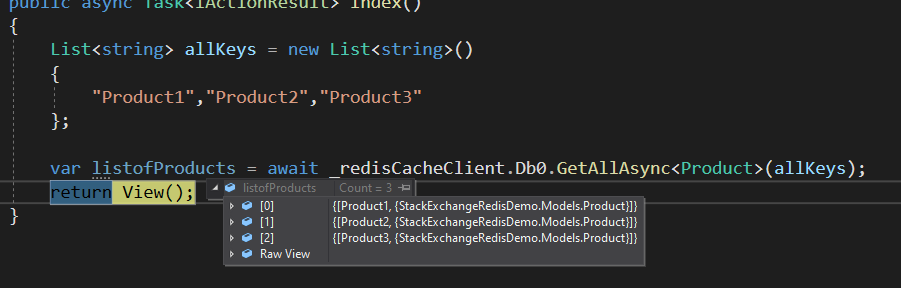
RemoveAsync 删除一个对象
public async Task<IActionResult> Index()
{
bool isRemoved = await _redisCacheClient.Db0.RemoveAsync("Product");
return View();
}
RemoveAllAsync 删除多个对象
public async Task<IActionResult> Index()
{
List<string> allKeys = new List<string>()
{
"Product1",
"Product2",
"Product3"
};
await _redisCacheClient.Db0.RemoveAllAsync(allKeys);
return View();
}
ExistsAsync 判断对象是否存在
public async Task<IActionResult> Index()
{
bool isExists = await _redisCacheClient.Db0.ExistsAsync("Product");
return View();
}
SearchKeysAsync 通配符搜索键
- "Product*" 搜索所有以 Product 开头的键
- "*Product*" 搜索所有包含 Product 的键
- "*Product" 搜索所有已 Product 结尾的键
public async Task<IActionResult> Index()
{
var listofkeys1 = await _redisCacheClient.Db0.SearchKeysAsync("Product*");
var listofkeys2 = await _redisCacheClient.Db0.SearchKeysAsync("*Product*");
var listofkeys3 = await _redisCacheClient.Db0.SearchKeysAsync("*Product");
return View();
}